Operating System - Learn Thread with C/C++, Python
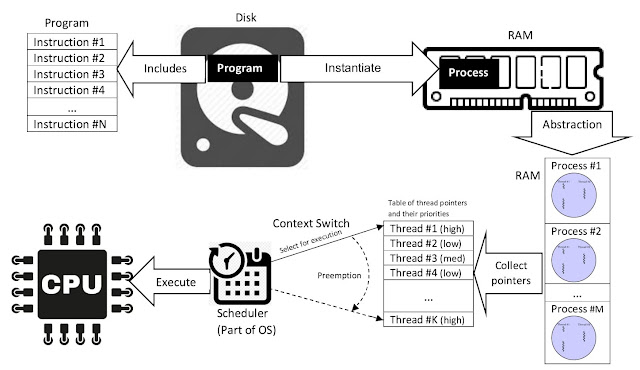
Learn Thread with C/C++, Python What is Thread ? Thread is the smallest sequence of programmed instructions that can be managed independently by a scheduler, which is typically a part of the operating system. Multiple threads can reside in a process, executing concurrently and sharing resources such as memory. While processes do not share resources, thread share resources in a process, and this leads to smaller overhead between context switching compared to processes. Program VS Process VS Thread Comparing process with thread Process Heavyweight unit of kernel scheduling due to their resources Resources include memory, file handles, sockets, device handles, windows and PCB Creating or destroying a process is relatively expensive as resources must be acquired or released. Thread Kernel Thread